Google Cloud Pub/Sub Receiver
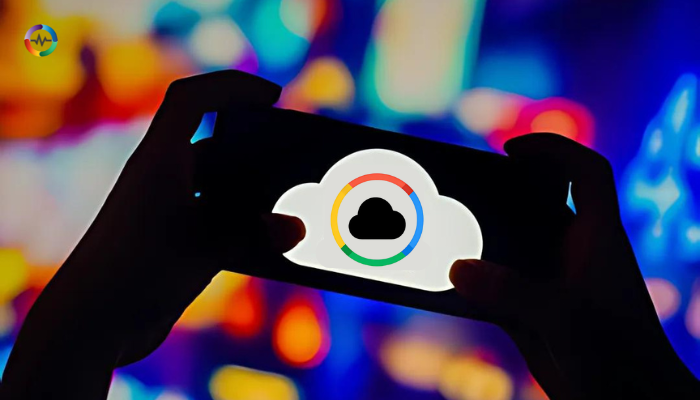
Welcome to our guide on the Google Cloud Pub/Sub Receiver. If you're a developer or an engineering manager looking to streamline your system's communication, you're in the right place.
Google Cloud Pub/Sub is a powerful tool that allows you to decouple your systems, ensuring seamless and efficient message exchanges.
What is Google Cloud Pub/Sub?
Google Cloud Pub/Sub is a messaging service that enables you to send and receive messages between independent applications.
Think of it as a reliable postal service for your data, making sure that messages get from point A to point B without any hiccups. This service is crucial for building scalable, flexible systems that can handle high volumes of data with ease.
Why Use Pub/Sub?
Pub/Sub helps achieve this by decoupling the producer (sender) and consumer (receiver) components of your applications.
This means that each part of your system can work independently, leading to better performance and easier maintenance.
Our guide will walk you through everything you need to know about creating and managing Pub/Sub resources, publishing and receiving messages, and handling common issues.
By the end of this blog, you'll have a solid understanding of how to leverage Google Cloud Pub/Sub to optimize your system's communication.
Ready to dive in? Let's get started with an overview of how to set up and manage your Pub/Sub resources effectively.
Creating and Managing Pub/Sub Resources
Before you can start sending and receiving messages, you need to understand the basics: topics and subscriptions.
Pub/Sub Topics are like bulletin boards where your messages get posted. When you publish a message, it goes to a topic.
Think of it as a central hub where all your messages live, waiting for subscribers to pick them up. Creating a topic is straightforward and can be done using the Google Cloud Console or API.
Creating a Pub/Sub Topic
To create a Pub/Sub topic:
- Using the Console:
- Go to the Google Cloud Console.
- Navigate to Pub/Sub.
- Click on "Create Topic."
- Enter a name for your topic and click "Create."
- Using the API:
- If you prefer using code, you can create a topic with a simple API call:
from google.cloud import pubsub_v1 |
Understanding Pub/Sub Subscriptions
Once you have your topics set up, you'll need subscriptions to pull messages. A Pub/Sub Subscription is like a subscription to a magazine. You subscribe to a topic, and you receive all the messages published to that topic.
This ensures that your applications get the data they need without missing anything.
Creating a Pub/Sub Subscription
To create a subscription:
- Using the Console:
- Go to the Pub/Sub section in the Google Cloud Console.
- Click on your topic.
- Select "Create Subscription."
- Enter a name for your subscription and configure the settings as needed.
- Click "Create."
- Using the API:
- Here’s how you can create a subscription via API:
from google.cloud import pubsub_v1 |
With your topics and subscriptions in place, you're all set to start publishing and receiving messages. In the next section, we’ll dive into how to publish messages to a Pub/Sub topic effectively.
Publishing Messages
Now that you have your topics and subscriptions set up, it’s time to start sending messages. Publishing messages to a Pub/Sub topic is straightforward and can be done using various methods.
Structure of a Pub/Sub Message
A Pub/Sub message consists of two main parts: data and attributes.
The data is the payload you want to send, while attributes are optional key-value pairs that can provide additional context about the message.
Methods to Publish Messages
You can publish messages to a Pub/Sub topic using the Google Cloud Console or the API. Here’s how:
Using the Console
- Go to the Google Cloud Console.
- Navigate to Pub/Sub and select your topic.
- Click on "Publish message."
- Enter your message data and any attributes.
- Click "Publish."
Using the API
To publish messages programmatically, you can use the Pub/Sub API. Here’s a quick example in Python:
from google.cloud import pubsub_v1 |
Practical Examples and Code Snippets
Here are a few practical examples to help you get started with publishing messages:
- Simple Message:
data = "Hello, Pub/Sub!".encode("utf-8") |
- Message with Attributes:
data = "Message with attributes".encode("utf-8") |
- Batching Messages:
for i in range(10): |
By following these methods, you can effectively publish messages to your Pub/Sub topics, ensuring your data is transmitted efficiently and reliably.
In the next section, we’ll explore how to receive and handle messages from your Pub/Sub subscriptions.
Receiving Messages
With your messages published, it's time to set up how you'll receive them.
Pub/Sub offers two delivery mechanisms: Pull and Push.
Understanding these methods and how to implement them is crucial for efficient message handling.
Pull vs Push Delivery Mechanisms
Pull Delivery: In pull delivery, your application initiates requests to the Pub/Sub service to retrieve messages. This method gives you control over when to receive messages and how many to process at a time.
Push Delivery: In push delivery, Pub/Sub automatically sends messages to a specified endpoint as they become available. This method is useful for real-time processing but requires your application to be always ready to handle incoming messages.
Pulling Messages from a Pub/Sub Subscription
For most applications, pulling messages is a flexible and common approach. Here’s how you can do it:
- Using the Console:
- Go to the Pub/Sub section in the Google Cloud Console.
- Select your subscription.
- Click on "Pull."
- Using the API:
- Here’s a simple example using Python:
from google.cloud import pubsub_v1 |
Using Pub/Sub PullSensor for Message Retrieval and Acknowledgment
The Pub/Sub PullSensor simplifies message retrieval and acknowledgment by managing these tasks for you.
This ensures that your application remains responsive and efficient.
Deferrable Mode for Asynchronous Message Pulling
Deferrable mode allows your application to handle message pulling asynchronously.
This mode is beneficial for applications that need to process messages without blocking other tasks.
Practical Examples and Code Snippets for Pulling Messages
Here are some practical examples to help you get started with pulling messages:
Simple Pull Example:
response = subscriber.pull(subscription_path, max_messages=10) |
- Handling Multiple Messages:
response = subscriber.pull(subscription_path, max_messages=10) |
- Asynchronous Pulling:
import asyncio |
By setting up efficient message retrieval, your application can handle incoming data seamlessly.
In the next section, we'll dive into how to handle and acknowledge these messages properly.
Handling Pub/Sub Messages
Once you’ve received your messages, the next step is to handle them effectively.
Proper message handling ensures that your system runs smoothly and that messages are processed and acknowledged correctly.
Acknowledging Receipt of Messages
Acknowledging messages is crucial to prevent them from being redelivered. When you pull a message from a Pub/Sub subscription, you need to acknowledge it to inform the service that the message has been received and processed.
Example in Python:
from google.cloud import pubsub_v1 |
This simple callback function acknowledges each message as it is received.
Handling Message Delivery Retries
Sometimes, messages might not be processed correctly the first time. Pub/Sub automatically retries message delivery until it receives an acknowledgment.
To handle these retries effectively, ensure your application can process duplicate messages or implement idempotency.
Idempotency Tip:
- Ensure that processing the same message multiple times does not produce different results. Use unique message IDs to track processed messages.
Ensuring Message Delivery and Acting on Messages
To ensure message delivery, it's essential to handle any errors that occur during processing. This involves logging errors and potentially implementing a retry mechanism within your application.
Example:
def callback(message): |
Practical Examples and Code Snippets
Here are a few practical examples to illustrate proper message handling:
- Basic Acknowledgment:
def callback(message): |
- Handling Duplicate Messages:
processed_messages = set() |
- Error Handling and Retries:
def callback(message): |
By following these examples and guidelines, you can ensure that your Pub/Sub messages are handled efficiently and reliably.
In the next section, we’ll explore how to manage your subscriptions and topics, including creating and deleting them.
Managing Subscriptions and Topics
Efficiently managing your Pub/Sub subscriptions and topics is crucial for maintaining a clean and organized messaging system.
This section will guide you through the processes of creating, deleting, and maintaining your subscriptions and topics.
Deleting a Pub/Sub Subscription
Over time, you may need to delete subscriptions that are no longer in use. This helps keep your system organized and ensures that unused subscriptions don’t consume unnecessary resources.
Using the Console
- Go to the Google Cloud Console.
- Navigate to Pub/Sub.
- Select the subscription you want to delete.
- Click on "Delete."
- Confirm the deletion.
Using the API
Here’s how you can delete a subscription using the Pub/Sub API in Python:
from google.cloud import pubsub_v1 |
Practical Examples and Code Snippets for Deleting Subscriptions
Deleting a Single Subscription:
from google.cloud import pubsub_v1 |
Batch Deleting Subscriptions:
subscription_ids = ["sub-id-1", "sub-id-2", "sub-id-3"] |
Deleting a Pub/Sub Topic
Similarly, you may need to delete topics that are no longer needed. This helps keep your system streamlined and efficient.
Using the Console
- Go to the Google Cloud Console.
- Navigate to Pub/Sub.
- Select the topic you want to delete.
- Click on "Delete."
- Confirm the deletion.
Using the API
Here’s how you can delete a topic using the Pub/Sub API in Python:
from google.cloud import pubsub_v1 |
Practical Examples and Code Snippets for Deleting Topics
Deleting a Single Topic:
from google.cloud import pubsub_v1 |
Batch Deleting Topics:
topic_ids = ["topic-id-1", "topic-id-2", "topic-id-3"] |
By following these steps and using the provided code snippets, you can effectively manage your Pub/Sub subscriptions and topics. Keeping your messaging infrastructure clean and well-maintained ensures optimal performance and resource utilization.
In the next section, we'll cover common issues and troubleshooting tips to help you manage your Pub/Sub environment more effectively.
Common Issues and Troubleshooting
Managing a Pub/Sub environment can come with its own set of challenges. This section will help you address common issues and provide troubleshooting tips to ensure your Pub/Sub system runs smoothly.
Ensuring Message Delivery to All Instances
One common issue is ensuring that all instances of your application receive the messages they need. This can be particularly challenging in a distributed system.
Tip: Use Durable Subscribers
- Durable subscribers ensure that messages are not lost if an instance goes down temporarily. This can be set up by creating subscriptions with proper acknowledgment handling.
Verification that All Instances Have Received Messages
To verify that all instances have received messages, you need a reliable tracking mechanism.
Tip: Implement Logging and Monitoring
- Use logging to track message receipt and acknowledgment.
- Implement monitoring tools like Google Cloud Monitoring to keep an eye on message flow and identify any anomalies.
Example:
def callback(message): |
Strategies for Handling Undelivered Messages
Sometimes, messages might not get delivered due to various reasons, such as network issues or application downtime.
Tip: Use Dead Letter Topics
- Configure dead letter topics to capture undelivered messages. This allows you to review and reprocess them as needed.
Example:
from google.cloud import pubsub_v1 |
Challenges and Solutions in Setting Up Reliable Message Receipt
Setting up reliable message receipt can be complex, but following best practices can help mitigate common issues.
Tip: Use Proper Acknowledgment and Error Handling
- Always acknowledge messages after processing to prevent re-delivery.
- Implement error handling to manage processing failures and retries effectively.
Example:
def callback(message): |
By implementing these strategies and following the provided examples, you can address common issues and ensure your Pub/Sub environment remains reliable and efficient.
In the next section, we'll summarize the key functions and management tips for using Pub/Sub effectively.
Conclusion
Google Cloud Pub/Sub is a powerful tool that enables efficient, scalable messaging for your applications. By understanding and implementing best practices for creating and managing Pub/Sub resources, publishing and receiving messages, and handling common issues, you can ensure your system remains robust and responsive.
While this guide focuses on the setup and use of Pub/Sub, it's also important to have effective monitoring and visualization tools in place to ensure your messaging infrastructure runs smoothly. OpenObserve (O2) offers comprehensive observability features, including powerful dashboards and alert configurations, which can enhance your ability to maintain the health and performance of your Pub/Sub setup.
For more information and to get started with OpenObserve, visit our website, check out our GitHub repository, or sign up here to start using OpenObserve today.
Author:
The OpenObserve Team comprises dedicated professionals committed to revolutionizing system observability through their innovative platform, OpenObserve. Dedicated to streamlining data observation and system monitoring, offering high performance and cost-effective solutions for diverse use cases.