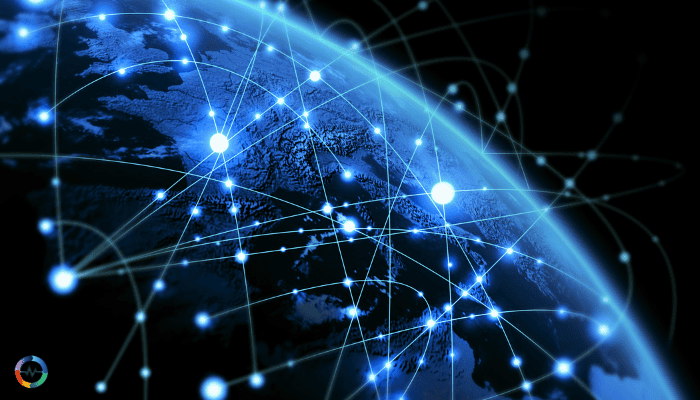
RabbitMQ is a powerhouse in the world of message-oriented middleware, providing a robust and reliable solution for message queuing. If you're diving into microservices or event-driven architecture, understanding how to receive messages efficiently with RabbitMQ is essential. This guide will walk you through the significance of RabbitMQ receivers and how to implement them in a Spring application, ensuring you get the most out of this powerful tool.
Why RabbitMQ?
In the modern software landscape, handling asynchronous communication between different components of your application is crucial. RabbitMQ shines by offering:
- Scalability: Easily handles increasing load and complex routing.
- Reliability: Guarantees message delivery with durability and acknowledgments.
- Flexibility: Supports multiple messaging protocols and can be integrated into various systems.
The Role of Message Receivers
At the heart of RabbitMQ's efficiency are its message receivers. These components ensure that messages are processed promptly and correctly, playing a critical role in maintaining the smooth operation of your applications. Whether you're building microservices or event-driven applications, understanding how to implement and optimize RabbitMQ receivers in a Spring application can significantly enhance your system's performance and reliability.
Getting Started
We'll start by setting up a basic Spring Boot project, integrating RabbitMQ, and configuring your application for seamless message processing. By the end of this guide, you'll have a solid foundation to efficiently receive and handle messages with RabbitMQ, making your applications more robust and scalable.
Let's get started with understanding the basics of RabbitMQ.
Understanding RabbitMQ
To effectively use RabbitMQ, it's important to grasp its core concepts and architecture. Let's break down the basics:
Basic Concepts of RabbitMQ
- Exchanges: These are responsible for routing messages to queues. RabbitMQ supports different types of exchanges (direct, topic, fanout, and headers), each serving a unique routing purpose.
- Queues: These are where messages are stored until they are processed. Queues ensure that messages are held in a safe place until a consumer is ready to handle them.
- Bindings: These define the relationship between exchanges and queues. A binding tells the exchange how to route messages to the appropriate queues.
Architecture of RabbitMQ
RabbitMQ follows a broker architecture, which means it acts as a middleman that manages the transmission of messages from producers to consumers. Here's a simplified view:
- Producer: Sends messages to an exchange.
- Exchange: Routes the messages to queues based on the binding rules.
- Queue: Stores messages until they are consumed.
- Consumer: Retrieves and processes messages from the queue.
RabbitMQ in Microservices and Event-Driven Architecture
In a microservices architecture, RabbitMQ helps decouple services, allowing them to communicate asynchronously. This decoupling enhances system scalability and resilience, as services can function independently. In event-driven architectures, RabbitMQ facilitates real-time processing and responsiveness by efficiently handling events and ensuring messages are delivered and processed without delays.
Why It Matters
Understanding these concepts is crucial as they form the foundation for building and managing RabbitMQ receivers in your Spring applications. With this knowledge, you're well on your way to effectively implementing RabbitMQ in your microservices or event-driven setups.
Next, we'll move on to setting up a basic Spring Boot project for RabbitMQ integration.
Creating a Basic Spring Application for RabbitMQ
Setting up a Spring Boot project to integrate RabbitMQ is straightforward and helps you manage message-driven applications efficiently. Here's how you can get started:
Setting Up a Spring Boot Project for RabbitMQ Integration
- Initialize the Project: Use Spring Initializr to create a new Spring Boot project. Make sure to include the necessary dependencies such as Spring Web, Spring AMQP, and any other dependencies your project might require.
- Add Dependencies: Your pom.xml or build.gradle file should include the RabbitMQ dependencies. For Maven, it looks like this:
<dependency> |
- Configure Application Properties: In your application.properties or application.yml file, configure the connection settings for RabbitMQ.
#properties spring.rabbitmq.host=localhost |
Configuring RabbitMQ Connection
- Create Configuration Class: Set up a configuration class to define the RabbitMQ components such as queues, exchanges, and bindings.
@Configuration |
Importance of Proper Configuration
Properly configuring your Spring application to connect with RabbitMQ ensures that your application can reliably send and receive messages. This setup is fundamental for building robust, scalable message-driven applications.
Next, we'll dive into implementing a RabbitMQ message receiver to handle incoming messages in your Spring application.
Implementing a RabbitMQ Message Receiver
Once you've set up your Spring Boot application and configured RabbitMQ, the next step is to implement a message receiver. This component is crucial for processing incoming messages efficiently.
The Concept of a Receiver in RabbitMQ
A receiver in RabbitMQ is a component that listens for messages on a specific queue and processes them. In a Spring application, this is typically done using a Plain Old Java Object (POJO) with methods annotated to handle messages.
Creating a Receiver Class as a POJO in Spring
- Define the Receiver Class: Create a simple POJO that will act as the message receiver.
@Component |
- Annotate the Class for Message Handling: Use the @RabbitListener annotation to specify the queue to listen to.
@RabbitListener(queues = "myQueue") |
Understanding the Role of the Receiver in Processing Messages
The receiver's role is to handle the business logic associated with the incoming messages. This could involve various tasks such as updating a database, calling another service, or triggering other processes within your application.
Example of a Complete Receiver Class
Here's a complete example of a receiver class in Spring that handles messages from RabbitMQ:
@Component |
With the receiver class in place, your Spring application is now set up to listen for and process messages from RabbitMQ. Next, we'll configure message listener containers to ensure our receiver works seamlessly within the Spring context.
Configuring Message Listener Containers
Message listener containers are essential for managing the lifecycle of message listeners in a Spring application. They handle the creation and destruction of listeners and ensure that messages are processed correctly.
Explanation of Message Listener Containers
A message listener container in Spring is responsible for listening to a RabbitMQ queue and invoking the appropriate listener methods when a message arrives. It abstracts the complexity of handling message acknowledgment, retries, and error handling, providing a robust way to manage message listeners.
Configuring a Message Listener Container for a RabbitMQ Receiver
Define the Configuration Class:
Create a configuration class to set up the listener container. This class will be annotated with @Configuration to indicate that it provides Spring beans.
@Configuration |
Configure the Connection Factory:
The connection factory is a crucial part of the configuration, as it establishes the connection to RabbitMQ.
@Bean |
Set Up the Listener Container:
Use the configured SimpleRabbitListenerContainerFactory to create a listener container that listens for messages on the specified queue.
@Bean |
Connecting Queues, Exchanges, and Bindings in Spring Configuration
To ensure that messages are correctly routed to your receiver, you need to configure queues, exchanges, and bindings in your Spring application.
Define the Queue:
@Bean |
Define the Exchange:
@Bean |
Define the Binding:
@Bean |
With these configurations, your Spring application is now set up to handle messages from RabbitMQ efficiently. The message listener container will manage the listeners, ensuring that messages are processed correctly and reliably.
Next, we’ll look at how to register listeners and handle messages.
Registering Listeners and Handling Messages
Now that we have configured the message listener container, the next step is to register listeners and handle the incoming messages. This is where we define the methods that will process the messages received from RabbitMQ.
The Process of Registering a Receiver as a Listener for Message Handling
In a Spring application, you can register a receiver as a listener using the @RabbitListener annotation. This annotation marks a method to be the target of a RabbitMQ message listener on the specified queue.
Define the Receiver Class:
Create a class to act as the message receiver. This class will contain methods annotated with @RabbitListener to handle incoming messages.
@Component |
Handling Different Types of Messages:
You can define multiple methods to handle different types of messages or messages from different queues. The @RabbitListener annotation allows specifying the queue(s) that each method should listen to.
@RabbitListener(queues = "anotherQueue") |
Using @RabbitListener with Advanced Options:
The @RabbitListener annotation provides several options for configuring the listener. For example, you can specify a container factory, set concurrency limits, and configure error handling.
@RabbitListener(queues = "myQueue", containerFactory = "rabbitListenerContainerFactory") |
Examples of Handling Different Types of Messages
Handling JSON Messages:
If your application receives JSON messages, you can use Spring’s MessageConverter to automatically convert the JSON payload into a Java object.
@RabbitListener(queues = "jsonQueue") |
Handling Messages with Headers:
You can also handle messages that include headers by using the Message class from the org.springframework.amqp.core package.
@RabbitListener(queues = "headerQueue") |
By registering listeners and defining methods to handle different types of messages, your Spring application can effectively process messages received from RabbitMQ. This setup ensures that your application can respond to various message formats and content, enhancing its flexibility and robustness.
In the next section, we’ll focus on testing the RabbitMQ receiver to ensure it processes messages correctly and efficiently.
Testing the RabbitMQ Receiver
Testing your RabbitMQ receiver is crucial to ensure that it processes messages correctly and efficiently. This involves sending test messages to RabbitMQ from your Spring application and verifying the receiver's functionality.
Sending Test Messages to RabbitMQ
Using RabbitTemplate:
Spring provides RabbitTemplate to send messages to RabbitMQ. You can use this template in your test cases to send test messages.
@SpringBootTest |
Configuring Test Properties:
Ensure your test configuration is set up correctly, including RabbitMQ connection properties and any necessary mocks or stubs.
spring.rabbitmq.host=localhost |
Verifying the Receiver's Ability to Process Messages
Writing Integration Tests:
Create integration tests to verify that the receiver processes the messages as expected. You can use the @SpringBootTest annotation to load the complete application context.
@SpringBootTest |
Mocking and Verifying:
Use mocking frameworks like Mockito to verify that the receiver's methods are called with the expected message content.
@ExtendWith(SpringExtension.class) |
Troubleshooting Common Issues in Message Receiving and Processing
- Connection Issues: Ensure RabbitMQ server is running and accessible from your Spring application. Check connection properties and firewall settings if there are connectivity problems.
- Message Conversion Errors: If you encounter message conversion errors, verify that the message format matches the expected input type in your receiver methods.
- Listener Configuration Problems: Ensure your listener configurations are correctly set up, including queue names, connection factories, and other properties.
- Handling Unprocessed Messages: If messages remain unprocessed, check for exceptions in the listener methods and ensure that the listener containers are properly configured and running.
By rigorously testing your RabbitMQ receiver, you can ensure that it handles messages accurately and reliably. This is a critical step in developing robust message-driven applications with RabbitMQ and Spring.
In the next section, we’ll discuss running and monitoring your Spring application to ensure optimal performance and reliability in message processing.
Running and Monitoring
Running and monitoring your Spring application with RabbitMQ integration is essential to ensure that your message receiver operates smoothly and efficiently.
This section covers how to run your application, monitor its performance, and implement best practices for production deployment.
Running the Spring Application to Receive Messages
Starting the Application:
Use your preferred method to start the Spring Boot application, whether through your IDE, command line, or deploying to a server.
./mvnw spring-boot:run |
Verifying the Setup:
Ensure that RabbitMQ is running and accessible. You can check RabbitMQ’s management interface to see if the queue is receiving messages and if the Spring application is connected.
spring: |
Monitoring and Logging Strategies
Using Spring Boot Actuator:
Spring Boot Actuator provides valuable insights into your application's health and metrics. Add Actuator to your dependencies and configure it for monitoring.
<dependency> |
Configure Actuator endpoints in your application.properties:
management.endpoints.web.exposure.include=health,info,metrics |
RabbitMQ Management Plugin:
RabbitMQ’s management plugin offers a web-based UI for monitoring queues, exchanges, and message rates. Enable the plugin to gain insights into your messaging setup.
rabbitmq-plugins enable rabbitmq_management |
Logging Strategies:
Implement structured logging to capture detailed information about message processing. Use libraries like Logback or SLF4J for efficient logging.
<dependency> |
Example of logging in your receiver:
@Component |
To ensure the continued reliability and efficiency of your RabbitMQ receivers, it’s essential to implement comprehensive monitoring and best practices. By leveraging robust tools and strategies, you can maintain optimal performance and swiftly address any issues that arise.
Integrating RabbitMQ with OpenObserve for Enhanced Monitoring
To ensure your RabbitMQ setup is running smoothly, it's essential to monitor its performance and health. Integrating RabbitMQ with OpenObserve (O2) allows you to leverage powerful dashboards and alert configurations for comprehensive observability.
- Install and Configure OpenObserve:
- Follow the installation guide to set up OpenObserve in your environment.
- Connect RabbitMQ to OpenObserve:
- Configure RabbitMQ to send metrics to OpenObserve. You can use RabbitMQ’s management plugin to expose metrics that OpenObserve can collect and visualize.
Example configuration:
openobserve: |
- Create Dashboards and Alerts in OpenObserve:
- Use OpenObserve’s intuitive UI to create custom dashboards that display key RabbitMQ metrics, such as queue length, message rates, and resource utilization. Set up alerts to notify you of any anomalies, ensuring you can respond quickly to potential issues.
Benefits of Integrating RabbitMQ with OpenObserve
- Real-Time Monitoring: Get immediate insights into your RabbitMQ performance with real-time metrics.
- Custom Dashboards: Create tailored dashboards to visualize important metrics specific to your RabbitMQ setup.
- Alerting: Configure alerts to stay informed about critical issues, helping you maintain a robust messaging system.
- Enhanced Troubleshooting: Leverage detailed metrics and logs for effective troubleshooting and optimization.
Conclusion
Incorporating RabbitMQ receivers into your Spring applications is a powerful way to manage and process messages efficiently. Through proper setup, robust monitoring, and adherence to best practices, you can achieve a scalable and resilient messaging system that supports your distributed architecture.
Setting up a RabbitMQ receiver ensures efficient message processing in your applications. Integrating RabbitMQ with OpenObserve (O2) enhances your ability to monitor and manage your messaging infrastructure effectively.
For more information and to get started with OpenObserve, visit our website, check out our GitHub repository, or sign up here to start using OpenObserve today.