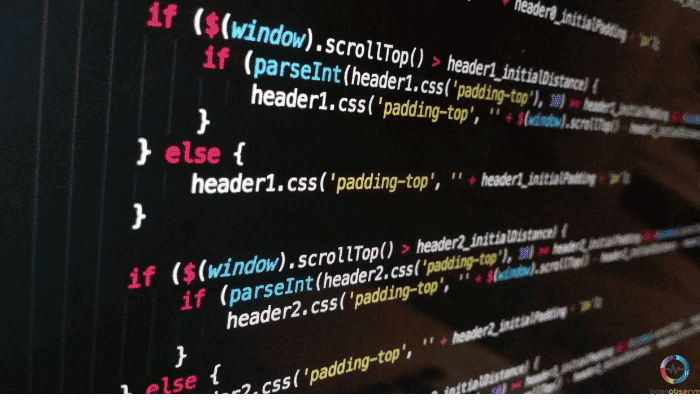
Introduction to JavaScript Logging
Role and Importance
The role and importance of JavaScript logging in web development and Agile methodologies are significant for enhancing the development process, ensuring code quality, and supporting Agile principles.
Here are the key points signifying the importance of JavaScript:
- Debugging and Error Tracking: JavaScript logging plays a crucial role in debugging applications by logging errors, warnings, and debug messages. It helps developers identify issues, trace the code flow, and inspect variables during development.
- Server Operations and Security: Logging in JavaScript is essential for server operations, security, intrusion detection, auditing, and business analytics. It provides insights into application performance, security threats, and operational issues, enabling proactive monitoring and response.
- Support for Agile Development: JavaScript's simplicity, flexibility, and support for continuous delivery align well with Agile principles. It enables rapid development, continuous testing, and deployment of valuable, working software, making it suitable for Agile methodologies.
- Enhanced Debugging Capabilities: JavaScript logging allows developers to log specific events, API responses, function calls, and stack traces. This detailed logging helps investigate issues, understand code behavior, and optimize application performance.
- Performance Profiling: Logging start and end times of operations in JavaScript helps in performance profiling, identifying bottlenecks, and optimizing code execution. It provides valuable insights into the time taken for specific operations and helps improve overall application performance.
JavaScript logging is a critical aspect of web development and Agile methodologies.
It enables developers to debug code effectively, monitor application performance, track errors, and support continuous improvement.
Evolution of JavaScript and its implications
JavaScript's evolution has been significant, transforming it from a simple scripting language into a versatile and powerful tool for web development.
The language has undergone various versions, each introducing new features and capabilities that have shaped its role in complex application development.
Here are the key implications of JavaScript's evolution for complex application development:
- Enhanced Functionality: JavaScript's evolution has led to the introduction of advanced features like asynchronous programming.
- Improved Developer Productivity: New versions of JavaScript have introduced syntax enhancements, modern language features, and powerful libraries and frameworks like React, Angular, and Vue.js.
- Support for Modern Web Development: JavaScript's evolution has enabled the development of modern web applications with rich user interfaces and real-time interactions.
The evolution of JavaScript has empowered developers to build sophisticated, interactive, and high-performance applications.
By leveraging the advancements in JavaScript and its ecosystem, developers can tackle the challenges of modern web development.
Get started for FREE with OpenObserve.
Client-side vs. Server-side Logging
Client-side logs are generated on the user's device, such as a web browser or mobile. They capture errors, warnings, and debug messages that occur during the execution of client-side code.
Server-side logs are generated on the server where the application is hosted.
They capture events, errors, and information related to server-side code execution, database interactions, and API calls.
Client-side logs provide insights into user-facing problems, while server-side logs help debug server-side issues.
Comparison: Client-side vs. Server-side Logging Features
Feature | Client-Side Logging | Server-Side Logging |
Data Collection | Direct access to user-specific data (cookies, URL parameters, user agent, referrer, IP address) | Limited data collection due to server-side restrictions |
Ease of Implementation | Easy to implement with standard practice and widely available expertise | Requires more setup and configuration |
Cost | Typically lower cost due to fewer vendor resources needed | Higher cost due to leveraging 3rd party vendor resources in the cloud |
Accuracy | Can be less accurate due to potential client-side issues | Generally more accurate due to reduced scope of data management |
Reliability | Less reliable due to potential client-side issues | More reliable due to reduced scope of data management |
Performance | Can impact application and device performance | Removes processing burden from client's device, improving performance |
Privacy | Can be less privacy-friendly due to data collection and tracking | Can be more privacy-friendly due to reduced data collection and tracking |
Tools and Frameworks | OpenObserve | OpenObserve |
The main challenges and limitations of accessing console logs in live environments are:
Client-Side Logging Challenges
- Lack of visibility into client-side errors
- Distributed logs across multiple clients
- Lack of local stack context for errors
- Inability to capture errors without user intervention
Server-Side Logging Advantages
- Centralized logging
- Comprehensive error tracking
- Automated log collection
Limitations of Console Logs in Live Environments
- Lack of persistence: Console logs only persist if explicitly saved or sent to a server.
- Potential performance impact: Excessive logging to the console can impact application performance.
- Difficulty managing and analyzing logs: Without log management tools, managing and analyzing large volumes of console logs can be challenging.
To overcome these challenges, it is recommended that applications in live environments be effectively monitored and debugged using a combination of client-side and server-side logging and log management tools.
Client-side logging can provide immediate feedback on user interactions and errors, while server-side logging offers a comprehensive application performance and security analysis.
The benefits of client-side logging and server-side logging are complementary and serve different purposes, providing immediate feedback and comprehensive analysis, respectively.
By combining client- and server-side logging, developers can better understand application behavior.
Logging Frameworks and Tools
Logging frameworks and tools are crucial in enhancing server-side error-logging capabilities by providing advanced features and simplifying the logging process.
Managing and analyzing large volumes of console logs can be challenging without log management tools. This includes tasks such as filtering, searching, and aggregating logs, which can be time-consuming and difficult to perform manually.
OpenObserve can help overcome the limitations of console logs in several ways:
- Persistent Storage: OpenObserve provides persistent storage for logs, ensuring that they are not lost even if the application is restarted or the console is closed.
- Efficient Logging: OpenObserve is designed for efficient logging, allowing for high-volume logging without impacting application performance.
- Advanced Log Management: OpenObserve provides advanced log management capabilities, including filtering, searching, and aggregating logs. This enables efficient analysis and troubleshooting of logs, making it easier to identify and resolve issues.
- Real-Time Insights: OpenObserve provides real-time insights into log data, enabling immediate detection and response to issues. This includes features such as alerting and dashboards for visualizing log data.
- Integration: OpenObserve integrates with various tools and frameworks, making it easy to incorporate into existing logging and monitoring workflows.
By integrating tools like OpenObserve, organizations can overcome the limitations of console logs and achieve more effective and efficient logging and troubleshooting in live environments.
About Us | Open Source Observability Platform for Logs, Metrics, Traces ...
Core JavaScript Logging Techniques
Overview of JavaScript’s Built-in Logging Methods and Message Levels
- JavaScript provides built-in logging methods like console.log(), console.info(), console.warn(), console.error(), and console.debug() for logging messages with different severity levels.
- Each method serves a distinct purpose and displays messages in the console in various forms and colors, helping developers differentiate between different log messages.
Get started for FREE with OpenObserve.
Effective Use of console.log(), console.info(), console. warn(), and console. error() for Varied Message Severities
- console.log(): Used for general logging and displaying informational messages.
- console.info(): Intended for informative messages without errors or warnings.
- console.warn(): Used for warning messages to alert developers about potential issues.
- console.error(): Specifically for logging error messages and highlighting critical issues in the code.
By appropriately utilizing these logging methods, developers can effectively communicate different types of messages and prioritize debugging efforts based on severity levels.
Advanced Techniques for Organizing Log Outputs using console. group() and console.groupEnd()
- console. group() and console.groupEnd() are used to group related log messages together, providing a structured and organized view of the console output.
- Grouping log messages helps categorize and organize information, making it easier to analyze and debug complex codebases with multiple log entries.
Performance Monitoring with console.time() and console.timeEnd() for Code Execution Timing
- console.time() and console.timeEnd() are used to measure the time taken for code execution between the two calls, allowing developers to monitor performance and identify bottlenecks in the code.
- By timing specific code blocks, developers can optimize performance, track algorithm efficiency, and improve overall application speed.
Error Management using try...catch...finally Syntax to Prevent Program Disruption.
- The try...catch...finally syntax is used to handle exceptions and errors in JavaScript code, preventing program disruption and enabling graceful error handling.
- By wrapping potentially error-prone code in a try block and handling exceptions in a catch block, developers can ensure that errors are caught and managed effectively, preventing crashes and improving application stability.
By mastering these core JavaScript logging techniques, developers can enhance their debugging skills, improve code quality, and streamline development.
Advanced Console Methods and Practical Examples
Here is a detail of the advanced console methods and practical examples:
Demonstrating Code Execution Timing, Message Grouping, and Error Handling
Measuring Code Execution Time:
javascript
console.time('myTimer');
// Some complex code here
console.timeEnd('myTimer');
The console.time() and console.timeEnd() methods allow you to measure the time taken for a block of code to execute. The label passed to both methods must match.
Grouping Console Messages:
javascript
console.group('User Actions');
console.log('User clicked button');
console.groupCollapsed('Detailed Info');
console.log('Button coordinates: x=100, y=200');
console.groupEnd();
console.groupEnd();
The console.group() and console.groupEnd() methods let you group related log messages together, creating a collapsible section in the console.
console.groupCollapsed() starts a group in a collapsed state.
Handling Errors with try...catch:
javascript
try {
// Some code that might throw an error
throw new Error('Something went wrong');
} catch (error) {
console.error('Error occurred:', error);
} finally {
console.log('Cleanup code executed');
}
The try...catch...finally syntax allows you to handle exceptions and errors, log the error message using console.error(), and execute cleanup code in the finally block.
Using console.table() for Visual Representation of Complex Data
javascript
const users = [
{ name: 'John Doe', age: 32, email: 'john@example.com' },
{ name: 'Jane Smith', age: 28, email: 'jane@example.com' },
{ name: 'Bob Johnson', age: 45, email: 'bob@example.com' },
];
console.table(users);
The console.table() method displays the provided data (an array or object) in a tabular format, making it easier to visualize and inspect complex data structures.
Real-Time Debugging with Pinned Expressions and Stack Tracing
Pinning Expressions:
javascript
let count = 0;
console.log('Count:', count);
console.log('Count:', ++count);
console.log('Count:', count);
You can pin expressions in the console by right-clicking on the log and selecting "Expand all" or "Expand recursively".
This allows you to monitor the value of the count variable in real time as it changes.
Stack Tracing with console.trace():
javascript
function a() {
b();
}
function b() {
c();
}
function c() {
console.trace('This is the trace');
}
a();
The console.trace() method outputs a stack trace to the console, helping you understand the call stack and the sequence of function invocations that led to the current point in the code.
Preserving Console Logs and Clearing the Console
Preserving Console Logs:
javascript
localStorage.setItem('consoleLog', console.log.bind(console));
// Later, you can retrieve and use the stored log function:
const storedLog = localStorage.getItem('consoleLog');
storedLog('This message will be preserved across page navigations');
By storing the console.log() function in the browser's localStorage, you can preserve log messages across page navigations and reuse the stored log function later.
Clearing the Console:
javascript
console.clear();
The console.clear() method clears the console, removing all previous log messages. In some browsers, it may also display a "Console was cleared" message.
These advanced console methods and practical examples demonstrate how you can leverage the power of the JavaScript console to enhance your debugging and development workflow.
By mastering these techniques, you can improve code quality, optimize performance, and streamline the debugging process.
Get started for FREE with OpenObserve.
Optimizing and Debugging with Console Tools
Techniques to Filter, Format, and Enhance Console Log Messages for Efficient Debugging:
- Utilize console.group() and console.groupEnd() to group related log messages, enhancing organization and readability.
- Apply CSS-based styling to log messages using format specifiers and CSS styles in console.log() for visual differentiation.
- Use console.table() to display complex data structures in a tabular format, improving data visualization and analysis.
Code Examples Illustrating Logging Methods, Message Formatting, and Console Utilities for DOM Inspection:
- Implement console.log(), console.info(), console.warn(), and console.error() for logging messages with varying severity levels.
- Utilize console.dir() for detailed object inspection and console.assert() for conditional logging based on boolean conditions.
- Employ console.time() and console.timeEnd() to measure code execution time and identify performance bottlenecks.
Tips for Including Context in Logs to Address Challenges of Logging Without Adequate Information:
- Use descriptive log messages with context-specific information to clarify the purpose and origin of each log entry.
- Incorporate relevant data, timestamps, and function names in log messages to facilitate tracing and debugging.
- Leverage console.trace() to generate stack traces and track the execution flow, aiding in identifying the source of errors and exceptions.
Introduction to Remote Logging Solutions like OpenObserve for Capturing Logs from Users’ Devices:
- Explore remote logging solutions like OpenObserve, which captures logs from real users' devices to provide insights into application behavior and performance.
- Utilize remote logging tools to monitor and analyze logs in real-time, enabling developers to diagnose issues, track user interactions, and improve overall application quality.
- Implement remote logging for efficient bug tracking, post-release debugging, and performance optimization, ensuring a seamless user experience and robust application performance.
By incorporating these techniques and tools into your debugging workflow, you can optimize console logging, enhance code analysis, and streamline the debugging process for efficient development and troubleshooting.
Best Practices for JavaScript Logging
Here are some best practices for effective JavaScript logging:
Recommendations for Color-Coding Logs, Grouping Messages, and Utilizing Console Methods
- Use console.log(), console.info(), console.warn(), and console.error() to log messages with varying severity levels, each with its color coding for quick visual differentiation.
- Group related log messages using console.group() and console.groupEnd() for better organization and readability.
- Employ console.table() to display complex data structures like arrays or objects in a tabular format, providing a clearer data view.
- Utilize console.time() and console.timeEnd() to measure the execution time of code blocks and identify performance bottlenecks.
Importance of Context in Error Logging and Strategies for Logging at Appropriate Levels
- Provide necessary context in log messages, such as the origin of the log, relevant data, timestamps, and function names, to facilitate tracing and debugging.
- Use descriptive log messages that are easily readable by humans and machines, enabling post-mortem debugging.
- Adopt a structured logging format like JSON to make log entries machine-readable while maintaining human readability.
- Log at appropriate levels based on the severity of the message, reserving console.error() for critical issues, console.warn() for warnings, and console.info() or console.log() for informational messages.
Considerations for Optimizing Logging Performance and Managing Log Data
- Avoid excessive logging to minimize performance impact on the application.
- Use a logging library like Winston or Bunyan that provides robust features for formatting, storing, and distributing logs.
- Configure the logging library to output logs to the desired destination, such as the terminal, filesystem, or a remote logging service.
- Implement log rotation and archiving strategies to manage log data efficiently and prevent storage issues.
By following these best practices, developers can leverage the power of JavaScript logging to enhance and streamline the development process.
Effective logging enables a better understanding of application behavior, faster issue resolution, and overall system reliability.
Conclusion and Future Directions
Summary
- Effective JavaScript logging is crucial for enhancing the reliability and stability of web applications.
- By leveraging the various logging methods and techniques, developers can gain valuable insights into application behavior, identify and resolve issues more efficiently, and ensure a smooth user experience.
Proficient logging practices enable proactive error management. They allow developers to catch and address issues early in the development cycle, reducing the impact of bugs in production environments.
Explore
- Developers should take advantage of the powerful features offered by browser developer tools, such as the console, to streamline their debugging workflow.
- Exploring logging frameworks and libraries like Winston or Bunyan can provide additional formatting, storing, and distributing capabilities, further enhancing the logging experience.
- Integrating with services like OpenObserve enables remote logging. Developers can capture logs from users' devices and gain insights into real-world application behavior, leading to faster bug resolution.
Final thoughts
- As web applications continue to grow in complexity, staying up-to-date with the latest logging techniques and best practices is essential for maintaining code quality and reliability.
- Developers should adopt a proactive approach to error management, incorporating logging as an integral part of their development workflow.
By continuously exploring new tools, techniques, and strategies for effective logging and error handling, developers can ensure that their web applications remain robust, scalable, and user-friendly in the face of evolving requirements and challenges.
In conclusion, proficient JavaScript logging is a fundamental skill for web developers, enabling them to build reliable, high-quality applications that meet the demands of modern web development.
Get started for FREE with OpenObserve.
By embracing the power of logging and staying committed to continuous improvement in error management practices, developers can contribute to the ongoing evolution and success of the web ecosystem.
Who are we?
We provide the simplest and most sophisticated open-source observability platform. Our flagship product, OpenObserve, gives you a single unified platform to collect, process, and visualize all your logs, metrics, and traces.
We are committed to building the best observability platform for developers and operators. We believe in open source and thrive on building a community around our products.
We are a remote-first company with a globally distributed team. We are headquartered in San Francisco, California.
Get in touch with us.
Let's talk
Send us an email at hello@openobserve.ai or connect with us: