Elasticsearch Match Query Usage and Examples
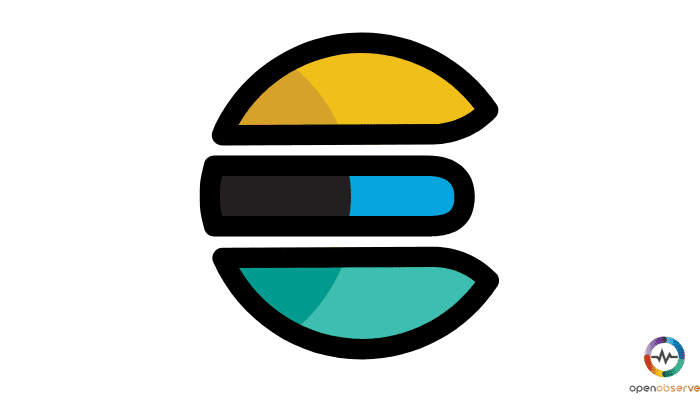
Introduction to Elasticsearch Match Query
Welcome to your guide on Elasticsearch Match Query, a cornerstone of effective search in your Elasticsearch toolkit. This blog will walk you through the essentials of using Match Query, a powerful tool designed to perform full-text searches with precision and flexibility.
What is an Elasticsearch Match Query?
Elasticsearch Match Query is a versatile search mechanism that allows you to perform both exact and fuzzy matches across various data types, including text, numbers, dates, and boolean values. It's the go-to method for searching through large datasets, ensuring you get relevant results quickly and efficiently.
For those looking to enhance their logging capabilities beyond Elasticsearch, OpenObserve offers a more streamlined, cost-effective solution. With its efficient handling of logs, metrics, and traces, OpenObserve can significantly reduce your storage costs and resource utilization. Check it out on GitHub.
How Does Match Query Work?
Think of Match Query as a diligent librarian who can find exactly what you're looking for, even if you're not quite sure how to spell it. It analyzes the search terms you provide, breaks them down into components, and then searches your data to find the best matches. Here’s a closer look at how it operates:
- Full-Text Search: Match Query is excellent for searching through text fields. It parses the input text into tokens (words or phrases) and searches for these tokens within your documents. This process ensures that even partial matches or variations of words can be found.
- Fuzzy Matching: One of the standout features of Match Query is its ability to handle typos and slight variations in search terms. By enabling fuzzy matching, you can ensure that minor spelling mistakes or variations in user input don't prevent relevant results from being found.
- Multi-Field Search: You can configure Match Query to search across multiple fields simultaneously. This is particularly useful when you have data spread across various attributes and need a comprehensive search solution.
Why Use Match Query?
Match Query is essential for any Elasticsearch setup because it provides a balance of precision and flexibility. Whether you need exact matches or want to account for user errors, Match Query delivers. It's especially useful in applications where search accuracy directly impacts user satisfaction, such as e-commerce sites, document repositories, and large-scale data analysis platforms.
Understanding Match Query
In this section, we'll delve into how Elasticsearch Match Query operates under the hood. By understanding its mechanisms and capabilities, you'll be better equipped to use it effectively in your applications.
How Match Query Analyzes and Searches Text
When you use a Match Query, Elasticsearch breaks down your input text into tokens—a process called text analysis. This involves several steps:
- Tokenization: The input text is split into individual terms or tokens. For instance, the phrase "quick brown fox" would be split into the tokens "quick," "brown," and "fox."
- Normalization: Tokens are converted to a standard form. This typically involves lowercasing all tokens to ensure case-insensitive search.
- Filtering: Common stopwords (like "and," "the," etc.) may be removed, depending on the analyzer used.
Once the text is analyzed, Elasticsearch searches for these tokens in the specified fields of your documents. This process allows for flexible and efficient full-text search capabilities.
Types of Searches Match Query Can Handle
Phrase Matching
Phrase matching is useful when you need to find documents containing an exact sequence of terms. For example, if you want to find the phrase "quick brown fox," you can set up a Match Query like this:
{ "query": { "match_phrase": { "description": "quick brown fox" } } }
This ensures that only documents containing the exact phrase "quick brown fox" in the specified order will be returned.
Proximity Matching
Proximity matching, or "slop" matching, allows you to find terms that are close to each other within a certain distance. This is useful for searching phrases where the word order might vary slightly. For example, to find "quick brown fox" with some flexibility in word order, you can use:
{ "query": { "match_phrase": { "description": { "query": "quick brown fox", "slop": 2 } } } }
Here, the slop parameter allows for up to two intervening words between "quick," "brown," and "fox," making the search more flexible.
Fuzzy Matching
Fuzzy matching helps in scenarios where there might be minor spelling errors or variations in the input text. For instance, if users might type "quik" instead of "quick," you can use a fuzzy match to catch these variations:
{ "query": { "match": { "description": { "query": "quik", "fuzziness": "AUTO" } } } }
The fuzziness parameter automatically adjusts to allow for a certain number of character changes, improving the chances of finding relevant matches despite minor errors.
By mastering these techniques, you can significantly enhance the search capabilities of your Elasticsearch implementations, ensuring that users find what they're looking for quickly and accurately.
Syntax and Example Usage
Now that we’ve covered the fundamentals and types of searches that Elasticsearch Match Query can handle, let’s dive into the syntax and how to construct these queries in different programming languages. We’ll start with a basic example and then see how it can be implemented in Python.
Basic Match Query Syntax
The syntax for a Match Query in Elasticsearch is straightforward. Here’s a simple example that searches for the term "text" in the "field":
{ "query": { "match": { "field": "text" } } }
This query tells Elasticsearch to look for documents where the "field" contains the term "text."
Constructing a Match Query in Python
Using Python to interact with Elasticsearch is common, especially with the help of the elasticsearch-py client. Here’s how you can construct and execute a basic Match Query using this library:
- Install the Elasticsearch Python Client: First, ensure you have the elasticsearch package installed
pip install elasticsearch
- Construct the Match Query:
from elasticsearch import Elasticsearch # Initialize the Elasticsearch client es = Elasticsearch() # Define the Match Query match_query = { "query": { "match": { "field": "text" } } } # Execute the query response = es.search(index="your_index", body=match_query) print(response)
This script connects to an Elasticsearch cluster, defines a Match Query searching for the term "text" in the "field," and then executes the query on the specified index. The results are printed out, showing the documents that match the search criteria.
For more advanced searches, you might want to include additional options like fuzziness or searching multiple fields.
Here’s an example that includes fuzzy matching and searches across multiple fields:
{ "query": { "multi_match": { "query": "text", "fields": ["field1", "field2"], "fuzziness": "AUTO" } } }
And here’s how you can implement this in Python:
# Define the advanced Match Query advanced_match_query = { "query": { "multi_match": { "query": "text", "fields": ["field1", "field2"], "fuzziness": "AUTO" } } } # Execute the advanced query advanced_response = es.search(index="your_index", body=advanced_match_query) print(advanced_response)
In this example, the query searches for the term "text" in both field1 and field2, using fuzziness to account for minor typos or variations.
If you find managing Elasticsearch queries cumbersome, OpenObserve simplifies this process with its intuitive interface and powerful search capabilities. It can replace Elasticsearch seamlessly for users who ingest data using APIs and perform searches. Get started quickly with OpenObserve by visiting here.
Important Parameters for Match Query
To leverage the full potential of Elasticsearch Match Query, it’s crucial to understand its key parameters. Each parameter plays a role in fine-tuning your search, making it more effective and tailored to your specific needs.
Let's break down these important parameters.
1. Query and Field (Both Required)
The core components of any Match Query are the query and field. The query parameter defines the search terms, while the field specifies which document field to search in. Without these, a Match Query cannot function.
Example:
{ "query": { "match": { "description": "Elasticsearch" } } }
2. Analyzer (Optional)
The analyzer parameter allows you to specify which analyzer to use for processing the text. Analyzers can tokenize text, convert it to lowercase, remove stopwords, and perform other text transformations.
Example:
{ "query": { "match": { "description": { "query": "Elasticsearch", "analyzer": "standard" } } } }
3. Fuzziness (Optional)
The fuzziness parameter handles typos and spelling variations, making your search more forgiving. It allows Elasticsearch to find results even if the search terms have minor errors.
Example:
{ "query": { "match": { "description": { "query": "Elastisearch", "fuzziness": "AUTO" } } } }
4. Operator (Optional)
The operator parameter controls the boolean logic used to include terms. It can be set to AND or OR, determining whether all terms (AND) or any term (OR) must match.
Example:
{ "query": { "match": { "description": { "query": "Elasticsearch query", "operator": "AND" } } } }
5. Minimum_Should_Match (Optional)
The minimum_should_match parameter specifies the minimum number of query terms that must match. This is useful for ensuring a certain level of relevance in the results.
Example:
{ "query": { "match": { "description": { "query": "Elasticsearch query matching", "minimum_should_match": "75%" } } } }
6. Zero_Terms_Query (Optional)
The zero_terms_query parameter configures the behavior when no terms remain after analysis. You can set it to none (return no documents) or all (return all documents).
Example:
{ "query": { "match": { "description": { "query": "", "zero_terms_query": "all" } } } }
By understanding and utilizing these parameters, you can refine your Match Queries to better meet your search needs. Whether you’re dealing with simple text searches or complex queries requiring typo tolerance and specific term matching rules, these parameters provide the flexibility needed to craft precise and efficient searches.
Here’s a comprehensive example combining multiple parameters:
{ "query": { "match": { "description": { "query": "Elasticsearch fuzzy query", "analyzer": "standard", "fuzziness": "AUTO", "operator": "AND", "minimum_should_match": "75%", "zero_terms_query": "all" } } } }
This query searches for "Elasticsearch fuzzy query" in the description field, using the standard analyzer. It includes typo tolerance, requires all terms to be present, mandates that 75% of the terms must match, and returns all documents if no terms remain after analysis.
By mastering these parameters, you can optimize your Elasticsearch Match Queries, ensuring they are robust, flexible, and highly effective for your specific use cases.
Setting up and managing these parameters in Elasticsearch can be intricate. OpenObserve, on the other hand, offers user-friendly configuration options that make handling logs and metrics straightforward and efficient. Explore how OpenObserve can simplify your observability needs by booking a demo on our website.
Controlling Search Precision
To maximize the effectiveness of your Elasticsearch Match Query, it's essential to understand how to control search precision.
One of the key ways to do this is by using the operator parameter, which fine-tunes the logical relationship between search terms. Let's explore how this works and see some examples in action.
Using the 'Operator' Parameter
The operator parameter in Elasticsearch Match Query defines how the search terms are combined to match documents. You can set this parameter to either AND or OR, which directly impacts the precision and recall of your search results.
- operator: "AND": All specified terms must be present in the document for it to be considered a match. This setting increases precision by ensuring that only documents containing all search terms are returned.
- operator: "OR": At least one of the specified terms must be present in the document. This setting increases recall by returning documents that contain any of the search terms, even if only one term matches.
For those dealing with complex search precision requirements, OpenObserve provides an alternative that simplifies these operations while maintaining accuracy and efficiency. Learn more about how OpenObserve can optimize your search operations on GitHub.
Examples Demonstrating 'AND' and 'OR' Operators
Let's look at examples to see the difference between these operators in practice.
Example 1: Using operator: "AND"
Suppose you want to find documents containing both "Elasticsearch" and "tutorial" in the content field. Here’s how you’d construct your query:
{ "query": { "match": { "content": { "query": "Elasticsearch tutorial", "operator": "AND" } } } }
In this case, only documents that contain both "Elasticsearch" and "tutorial" in the content field will be returned. This ensures higher precision, as the results are more specific to documents discussing both topics.
Example 2: Using operator: "OR"
Now, let’s say you want to find documents that contain either "Elasticsearch" or "tutorial" in the content field. Here’s the query with the operator set to OR:
{ "query": { "match": { "content": { "query": "Elasticsearch tutorial", "operator": "OR" } } } }
Practical Considerations
When deciding between AND and OR, consider the following:
- Use AND when you need precise results and are sure that documents should contain all specified terms. This is particularly useful for technical documentation, research papers, or detailed guides where specificity is crucial.
- Use OR when you want to broaden your search and are looking for any documents related to any of the terms. This is useful for general information retrieval, exploratory searches, or when building a comprehensive database of related topics.
Implementing Fuzziness in Searches
Fuzziness in Elasticsearch Match Query allows for approximate matching, making your searches more flexible and tolerant of minor errors. This section will explain how fuzziness works and provide examples to illustrate its use.
Explanation of Fuzziness in Match Query
Fuzziness is a feature in Elasticsearch that enables the Match Query to handle variations in the search terms. This is particularly useful for dealing with typos, spelling errors, or different word forms that might occur in user input.
By allowing a certain degree of variation, fuzziness ensures that your search can still find relevant documents even when the search terms aren't exact matches.
Fuzziness works by calculating the Levenshtein distance, which measures the number of single-character edits (insertions, deletions, or substitutions) needed to change one word into another.
Managing fuzziness in search queries can sometimes be challenging with Elasticsearch.
OpenObserve offers enhanced search capabilities that include efficient fuzzy matching, making your search operations smoother. Start using OpenObserve today by visiting our website.
Example Showing How to Use 'Fuzziness' Setting
Let’s see how you can implement fuzziness in your Match Query to handle similar terms or minor misspellings.
Example: Basic Fuzzy Match
Suppose you want to search for documents containing the word "connection" in the description field, but you want the search to be tolerant of minor spelling mistakes like "conection" or "connecion." Here’s how you’d set up the query with fuzziness:
{ "query": { "match": { "description": { "query": "connection", "fuzziness": "AUTO" } } } }
In this query, the fuzziness parameter is set to AUTO, allowing Elasticsearch to determine the appropriate level of fuzziness based on the length of the search term. This setting will catch minor spelling variations and return relevant results even if the input term is slightly off.
Example: Manual Fuzziness Level
You can also set the fuzziness level manually to control the degree of variation allowed. Here’s an example where the fuzziness level is explicitly set to 2, allowing up to two character changes:
{ "query": { "match": { "description": { "query": "connection", "fuzziness": 2 } } } }
By setting fuzziness to 2, this query will find documents containing terms that are within two edits of "connection," such as "connecion" or "connction."
Utilizing Minimum Should Match
The minimum_should_match parameter in Elasticsearch Match Query provides another layer of control by specifying the minimum number of terms that must match for a document to be considered relevant.
This section will discuss how this parameter influences the required match count and provide examples of its usage.
How 'Minimum Should Match' Influences Match Count
The minimum_should_match parameter allows you to define how many of the search terms need to match in a document. This is particularly useful for long queries where you might want to ensure that a certain proportion of the terms are present in the search results.
By setting this parameter, you can fine-tune the balance between precision and recall in your search results.
- Exact Numbers: You can specify an exact number of terms that must match.
- Percentages: You can define the minimum number of matching terms as a percentage of the total terms.
- Combined Criteria: You can combine exact numbers and percentages for more complex requirements.
Example Queries Illustrating 'Minimum Should Match'
Example: Exact Number of Matches
If you want to ensure that at least three terms in the query "Elasticsearch fuzzy query matching" are present in the description field, you can set minimum_should_match to 3:
{ "query": { "match": { "description": { "query": "Elasticsearch fuzzy query matching", "minimum_should_match": 3 } } } }
This query will return documents that contain at least three of the terms from the search query.
Example: Percentage of Matches
You can also specify minimum_should_match as a percentage. For instance, to require that 75% of the terms match, you can use:
{ "query": { "match": { "description": { "query": "Elasticsearch fuzzy query matching", "minimum_should_match": "75%" } } } }
This query ensures that at least 75% of the terms in the search query are present in the document.
Example: Combined Criteria
You can combine exact numbers and percentages for more complex requirements. For example, to require either 75% of the terms or a minimum of three terms (whichever is greater), you can use:
{ "query": { "match": { "description": { "query": "Elasticsearch fuzzy query matching", "minimum_should_match": "3<75%" } } } }
This query ensures that at least three terms or 75% of the terms (whichever is higher) must match.
Practical Uses of Minimum Should Match
The minimum_should_match parameter is especially useful in scenarios where:
- User Input: Users may enter queries with multiple terms, and you want to ensure that most, if not all, terms are present in the results to maintain relevance.
- Long Queries: When dealing with long queries, you can use minimum_should_match to ensure that a significant portion of the query terms match, improving the relevance of the results.
- Controlled Flexibility: This parameter provides controlled flexibility, allowing for a balance between strict and loose matching criteria based on the query requirements.
For a more streamlined approach to handling match criteria, OpenObserve simplifies these configurations with its robust platform. It’s designed to handle large-scale log data with ease. Learn more and sign up for a free trial on the OpenObserve website.
Performance Considerations
1. Tips on optimizing the performance of match queries, particularly in full-text search applications.
2. Recommendations for selecting the appropriate parameters and query types based on the use case.
Optimizing the performance of Elasticsearch Match Queries is crucial, especially when dealing with full-text search applications that handle large volumes of data.
Here are some tips and recommendations to help you get the most out of your search queries while maintaining efficiency.
Tips on Optimizing the Performance of Match Queries
- Use Appropriate Analyzers:
- Tip: Choose the right analyzers for your data to ensure efficient text processing and accurate search results.
- Example: For English text, using the standard analyzer can help balance performance and accuracy by handling stopwords, stemming, and tokenization effectively.
- Limit the Number of Fields:
- Tip: Avoid searching across too many fields simultaneously, as this can slow down performance. Focus on the most relevant fields.
- Example: Instead of querying multiple fields unnecessarily, target specific fields that are more likely to contain the relevant information.
- Control Fuzziness:
- Tip: Use fuzziness judiciously, as it can increase query complexity. Set appropriate fuzziness levels to balance flexibility and performance.
- Example: Limit fuzziness to a reasonable level, such as fuzziness: "AUTO" or a specific number like 1, to prevent excessive resource consumption.
- Leverage Filters:
- Tip: Use filters to pre-process queries and reduce the amount of data Elasticsearch needs to analyze. Filters are faster than queries because they do not affect scoring.
- Example: Apply term filters to narrow down the dataset before running full-text queries.
- Index Optimization:
- Tip: Optimize your Elasticsearch index settings to improve query performance. This includes configuring the appropriate number of shards and replicas based on your data size and query load.
- Example: Use the _forcemerge API to optimize indices by reducing the number of segments, which can speed up search performance.
- Use Caching:
- Tip: Take advantage of Elasticsearch’s caching mechanisms to store frequently accessed query results, reducing the need to repeatedly compute them.
- Example: Enable request caching for heavy queries that are run frequently with similar parameters.
Optimizing Elasticsearch performance can be resource-intensive. OpenObserve is built to handle high-performance requirements efficiently, making it a superior alternative for log management. Discover how OpenObserve can enhance your system's performance by visiting our website or checking out our GitHub page.
Conclusion
In the realm of Elasticsearch, mastering Match Query is essential for building robust and efficient search functionalities. By understanding its core components and learning to fine-tune parameters like fuzziness, operator, and minimum_should_match, you can create powerful, flexible, and accurate search experiences.
We’ve explored the fundamentals, practical applications, and performance optimization tips to help you get the most out of Match Query. Whether you’re handling simple text searches or complex full-text search scenarios, the knowledge shared here equips you to tackle various challenges with confidence.
Remember, the key to effective search lies in continuous refinement. Regularly monitor your queries, adjust parameters based on real-world usage, and always strive for that perfect balance between precision and recall.
Author:
The OpenObserve Team comprises dedicated professionals committed to revolutionizing system observability through their innovative platform, OpenObserve. Dedicated to streamlining data observation and system monitoring, offering high performance and cost-effective solutions for diverse use cases.