Getting Started with Logging in Node.js
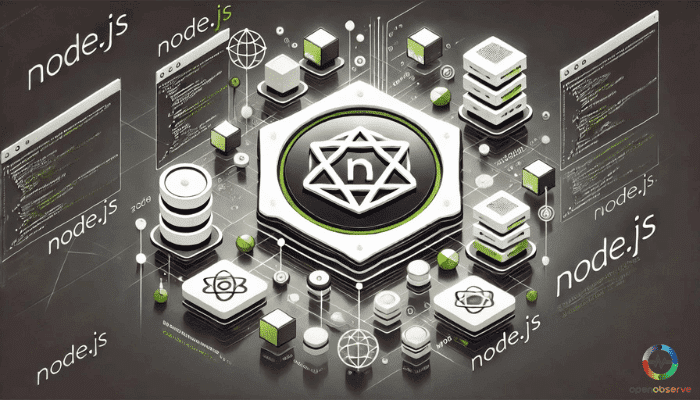
Introduction to Logging in Node.js
Logging is a crucial aspect of the application lifecycle, providing insights into the internal workings and behavior of your application. Whether you're debugging issues, tracking performance, or auditing user actions, effective logging practices can make a significant difference. In Node.js, logging can range from simple console statements to sophisticated logging libraries that offer advanced features and integrations.
In this guide, we'll cover everything you need to know about logging in Node.js, from beginner to advanced techniques. You'll learn about the different logging levels, when to use them, and how to implement them effectively. By the end of this guide, you'll be equipped with the knowledge to improve your application's observability and maintainability through effective logging practices.
Why Logging is Important in the Application Lifecycle
Logging serves multiple purposes throughout the application lifecycle:
- Debugging: Logs help you understand what went wrong when an error occurs, providing context and details that can be crucial for identifying the root cause.
- Performance Monitoring: By tracking execution times and resource usage, logs can highlight performance bottlenecks and inefficiencies.
- Security Auditing: Logs can record user actions and system events, helping to detect and investigate suspicious activities.
- Operational Insights: Logs provide a historical record of application behavior, useful for troubleshooting issues and optimizing performance.
Quick Overview of Logging Techniques in Node.js
Node.js offers several methods for logging, ranging from built-in console logging to more advanced logging libraries:
- Console Logging: Simple and straightforward, using console.log(), console.info(), console.warn(), and console.error() for different logging levels.
- Logging Libraries: Tools like Winston provide advanced features such as custom log levels, multiple transports, and structured logging.
- Log Management Tools: For large-scale applications, integrating with log management and aggregation tools like Papertrail can centralize logs and simplify analysis.
Distinguishing Between Logging Levels
Effective logging requires understanding and using different logging levels appropriately:
- Info: General informational messages about application progress or state changes.
- Warn: Potentially harmful situations that are not errors but might require attention.
- Error: Error events that might still allow the application to continue running.
- Debug: Detailed information for diagnosing problems, typically used in development.
- Trace: Finer-grained informational events than debug, often including detailed context.
Understanding these levels and when to use them can help you maintain clean and meaningful logs that provide valuable insights without overwhelming you with noise.
By mastering these logging techniques, you can ensure that your Node.js application is robust, maintainable, and easy to debug. In the following sections, we'll dive deeper into each aspect of logging in Node.js, starting with the basics of Node.js built-in logging methods.
Node.js Built-in Logging
Node.js provides built-in logging capabilities through its console module, which is straightforward and easy to use. While these methods are not suitable for production logging due to their limitations, they are a good starting point for understanding basic logging in Node.js.
Introduction to Node.js Console Logger
The console module in Node.js offers several methods for logging messages to the console:
- console.log(): Used for general logging of information.
- console.info(): Similar to console.log(), typically used for informational messages.
- console.warn(): Used for logging warning messages.
- console.error(): Used for logging error messages.
These methods are simple to use and provide a quick way to output messages to the console.
Differences and Use Cases for console.log(), console.info(), console.warn(), and console.error()
Each logging method serves a different purpose:
- console.log(): Used for general information and debugging messages.
console.log("Server started on port 3000");
- console.info(): Used for informational messages, often similar to console.log().
console.info("User logged in successfully");
- console.warn(): Used to indicate potential issues that are not critical.
console.warn("Disk space is running low");
- console.error(): Used for error messages and exceptions.
console.error("Failed to connect to the database", err);
Limitations of Built-in Logging
While these built-in methods are handy, they come with limitations:
- No Log Levels: Built-in logging methods do not support log levels, making it hard to differentiate between different types of logs.
- Unstructured Logs: Logs are plain text and lack structure, making them difficult to parse and analyze.
- No Centralized Management: Built-in logs are scattered and not centralized, complicating log management.
- Performance Impact: Extensive logging using console methods can affect the performance of your application.
To overcome these limitations, it's essential to explore more advanced logging libraries like Winston and OpenObserve.
Node.js Logging with Winston Library
For robust logging in Node.js, Winston is a go-to library, offering extensive features that surpass the built-in console methods. Winston's flexibility and support for multiple transports make it an ideal choice for comprehensive logging needs.
Benefits of Using Winston
- Custom Log Levels: Define and use custom log levels to categorize and manage logs effectively.
- Multiple Transports: Log to various destinations such as files, databases, or remote services simultaneously.
- Structured Logging: Format logs in JSON or other structured formats, making them easier to parse and analyze.
- Timestamping: Automatically include timestamps in each log entry for better traceability.
Example Configuration
Here's a simple setup to get you started with Winston:
const winston = require('winston'); const logger = winston.createLogger({ level: 'info', format: winston.format.json(), transports: [ new winston.transports.Console(), new winston.transports.File({ filename: 'combined.log' }) ] }); logger.info('Hello, Winston!');
Integrating Winston with OpenObserve
While OpenObserve's documentation primarily covers Pino, you can adapt similar steps to send Winston logs to OpenObserve. This involves using HTTP transport to send logs to OpenObserve's endpoint.
Step-by-Step Integration:
- Install Required Packages: First, ensure you have winston and a package to send HTTP requests, such as axios.
npm install winston axios
<strong>2. Configure Winston to Send Logs to OpenObserve:</strong> Create a custom Winston transport that sends logs to OpenObserve via HTTP.
const winston = require('winston'); const axios = require('axios'); const openObserveTransport = new winston.transports.Stream({ stream: { write: async (message) => { try { await axios.post('https://your-openobserve-endpoint/logs', { log: message, apiKey: 'your-api-key' // Replace with your actual OpenObserve API key }); } catch (error) { console.error('Error sending log to OpenObserve', error); } } } }); const logger = winston.createLogger({ level: 'info', format: winston.format.json(), transports: [ openObserveTransport, new winston.transports.Console() ] }); logger.info('Hello, OpenObserve!');
By following these steps, you can seamlessly integrate Winston with OpenObserve, allowing you to leverage advanced log management and analysis features provided by OpenObserve.
Ready to enhance your Node.js logging strategy? Sign up for a free trial of OpenObserve, explore our GitHub repository, or book a demo today.
Node.js Logging Best Practices
Adhering to best practices in logging ensures that your logs are useful, secure, and maintainable. Here are some essential guidelines to follow when implementing logging in your Node.js applications.
Avoid Using console.log() for Production Logs
While console.log() is useful for debugging during development, it is not suitable for production environments. It lacks features such as log levels, structured logging, and the ability to log to multiple destinations.
Implement Structured Logging
Structured logging involves formatting logs as structured data, typically in JSON format. This makes it easier to parse, search, and analyze logs.
const winston = require('winston'); const logger = winston.createLogger({ level: 'info', format: winston.format.json(), transports: [ new winston.transports.Console(), new winston.transports.File({ filename: 'app.log' }) ] }); logger.info({ message: 'User logged in', userId: 1234 });
Add Context to Your Logs
Including context in your logs helps in understanding the conditions under which an event occurred. This can include user IDs, request IDs, session IDs, and other relevant metadata.
logger.info({ message: 'User updated profile', userId: 1234, changes: { email: 'new@example.com' } });
Avoid Logging Sensitive Information
Ensure that sensitive information, such as passwords, credit card numbers, and personal identification numbers, is never logged. Mask or exclude sensitive data from your logs.
Automatically Log Uncaught Exceptions and Unhandled Promise Rejections
Capturing unhandled errors is crucial for diagnosing issues that cause your application to crash. Use process-level handlers to log these events.
process.on('uncaughtException', (err) => { logger.error('Uncaught Exception:', err); }); process.on('unhandledRejection', (reason, promise) => { logger.error('Unhandled Rejection:', reason); });
Log Rotation and Retention Policies
Implement log rotation to prevent log files from growing indefinitely. Configure log retention policies to keep logs for a reasonable period.
const DailyRotateFile = require('winston-daily-rotate-file');
const logger = winston.createLogger({ level: 'info', format: winston.format.json(), transports: [ new winston.transports.Console(), new DailyRotateFile({ filename: 'application-%DATE%.log', datePattern: 'YYYY-MM-DD', maxFiles: '14d' }) ] });
Leveraging OpenObserve for Advanced Log Management
Integrate your logging system with OpenObserve to benefit from advanced log management features. OpenObserve can provide centralized log aggregation, real-time analytics, and powerful visualization tools, enhancing your ability to monitor and troubleshoot your applications effectively.
Ready to take your logging to the next level? Sign up for a free trial of OpenObserve, explore our GitHub repository, or book a demo today.
Advanced Node.js Logging Techniques
For more sophisticated logging needs, you can leverage advanced techniques to enhance your logging capabilities. These techniques provide greater control and flexibility, enabling you to capture detailed information and handle complex logging requirements.
Using the debug Module for Granular Logging Control
The debug module allows you to enable or disable logging dynamically based on environment variables. This is useful for controlling the verbosity of your logs without changing your code.
const debug = require('debug')('app'); debug('This is a debug message');
To enable debug messages, set the DEBUG environment variable:
DEBUG=app node yourapp.js
Understanding Log Levels
Implementing log levels helps categorize the severity of log messages. Common log levels include:
- fatal: Critical errors causing application shutdown
- error: Runtime errors or unexpected conditions
- warn: Non-critical issues that could become problems
- info: General operational messages about application behavior
- debug: Detailed information for diagnosing issues
- trace: The most detailed level, including fine-grained information
logger.log('error', 'This is an error message'); logger.log('warn', 'This is a warning message'); logger.log('info', 'This is an informational message'); logger.log('debug', 'This is a debug message');
Implementing File Logging with the fs Module
For persistent logging, write log messages to a file using the fs module. This approach is useful for environments where external logging libraries are not an option.
const fs = require('fs'); function logToFile(message) { fs.appendFile('application.log', `${new Date().toISOString()} - ${message}\n`, (err) => { if (err) throw err; }); } logToFile('Application started');
Extending Logger Functionality to Other JavaScript Files
Create a custom logger module and reuse it across different parts of your application. This promotes consistency and makes it easier to manage your logging configuration.
// logger.js const winston = require('winston'); const logger = winston.createLogger({ level: 'info', format: winston.format.json(), transports: [ new winston.transports.Console(), new winston.transports.File({ filename: 'app.log' }) ] }); module.exports = logger; // In other files const logger = require('./logger'); logger.info('This is a log message from another file');
Advanced Log Management with OpenObserve
For comprehensive log management, integrate your Node.js application with OpenObserve. OpenObserve supports advanced features such as real-time log streaming, centralized log aggregation, and powerful analytics, enabling you to gain deeper insights into your application's behavior.
Setting Up OpenObserve with Node.js
To send logs from your Node.js application to OpenObserve, follow these steps:
- Install the OpenObserve SDK:
npm install openobserve-sdk
- Configure OpenObserve in Your Application:
const OpenObserve = require('openobserve-sdk'); const logger = new OpenObserve.Logger({ endpoint: 'https://openobserve.yourdomain.com', apiKey: 'your-api-key' }); logger.info('Node.js application started');
Integrating OpenObserve allows you to leverage its robust logging capabilities, providing real-time visibility into your application and enhancing your ability to monitor and troubleshoot effectively.
Ready to optimize your logging setup? Sign up for a free trial of OpenObserve, explore our GitHub repository, or book a demo today.
Log Management and Aggregation
The Importance of Centralizing Logs
Centralizing your logs is crucial for efficient troubleshooting and performance tracking. A unified log management system allows you to aggregate logs from various sources, making it easier to analyze and correlate data. This is particularly important in complex environments where multiple applications and services generate logs.
Introduction to OpenObserve for Log Management
OpenObserve is a powerful log management tool that offers advanced features for centralized log aggregation, real-time streaming, and in-depth analysis. By integrating OpenObserve into your Node.js logging setup, you can enhance your ability to monitor, troubleshoot, and optimize your application.
Setting Up OpenObserve for Log Aggregation
- Install OpenObserve SDK:
npm install openobserve-sdk
- Configure OpenObserve in Your Application::
const OpenObserve = require('openobserve-sdk'); const logger = new OpenObserve.Logger({ endpoint: 'https://openobserve.yourdomain.com', apiKey: 'your-api-key' }); logger.info('Centralized logging with OpenObserve initialized');
Benefits of Using OpenObserve for Log Aggregation
- Real-Time Log Streaming: Monitor logs in real-time to quickly identify and respond to issues as they occur.
- Centralized Log Storage: Aggregate logs from multiple sources into a single, unified view, simplifying log analysis.
- Advanced Analytics and Visualization: Utilize OpenObserve's powerful analytics tools to gain deeper insights into your log data, enabling more effective troubleshooting and performance optimization.
- Integration with Other Tools: Seamlessly integrate OpenObserve with other observability tools, enhancing your overall monitoring and observability strategy.
Example of Setting Up Log Aggregation
Here’s how you can set up OpenObserve for centralized log aggregation in your Node.js application:
- Configure Your Application:
const winston = require('winston'); const OpenObserveTransport = require('openobserve-sdk/transport'); const logger = winston.createLogger({ level: 'info', format: winston.format.json(), transports: [ new winston.transports.Console(), new OpenObserveTransport({ endpoint: 'https://openobserve.yourdomain.com', apiKey: 'your-api-key' }) ] }); logger.info('Log aggregation with OpenObserve setup complete');
- Verify Log Aggregation:
- Ensure that logs are being sent to OpenObserve by checking the OpenObserve dashboard.
- Use OpenObserve's query and analytics features to analyze your logs and gain insights.
By transitioning to OpenObserve, you can enhance your log management strategy and gain more actionable insights from your logs.
Ready to upgrade your log management? Sign up for a free trial of OpenObserve, explore our GitHub repository, or book a demo today.
Conclusion
Effective logging is crucial for maintaining the health and performance of your Node.js applications. By implementing robust logging practices and leveraging powerful tools like OpenObserve, you can gain deeper insights into your application's behavior, quickly identify and resolve issues, and ensure optimal performance.
OpenObserve offers advanced features for log aggregation, real-time streaming, and comprehensive analytics, making it an ideal choice for enhancing your Node.js logging strategy. Whether you're looking to replace basic logging methods or upgrade from existing log management tools, OpenObserve provides the capabilities you need for efficient and effective log management.
Ready to upgrade your log management? Sign up for a free trial of OpenObserve, explore our GitHub repository, or book a demo today.
Author:
The OpenObserve Team comprises dedicated professionals committed to revolutionizing system observability through their innovative platform, OpenObserve. Dedicated to streamlining data observation and system monitoring, offering high performance and cost-effective solutions for diverse use cases.