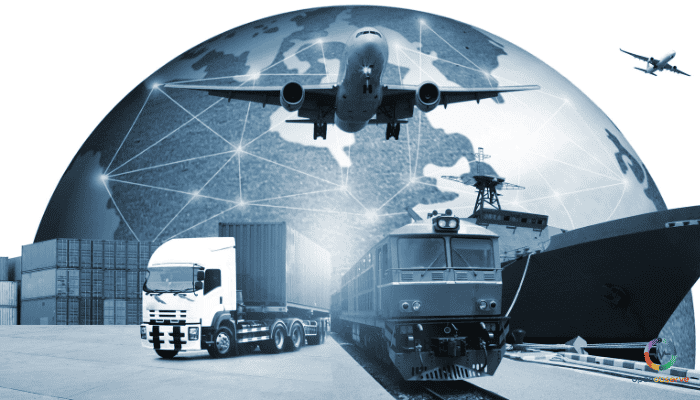
Tracing issues in a microservices architecture can feel like finding a needle in a haystack. But with Istio tracing, tracking down performance bottlenecks and understanding service-to-service communication becomes much easier. Whether you're a developer, DevOps engineer, or engineering manager, distributed tracing is your key to gaining visibility into how your microservices interact—without the guesswork.
In this guide, we'll explore Istio tracing, how Istio’s Envoy proxy generates and propagates tracing headers, and how you can configure and customise tracing for your microservices. By the end, you'll be equipped to implement tracing in your environment with confidence.
Ready to roll? Let’s get into the nuts and bolts of how Istio tracing works and how you can set it up.
Overview of Distributed Tracing with Istio
In the world of microservices, figuring out what went wrong can feel like putting together a puzzle where the pieces are scattered across multiple services. That’s where distributed tracing comes in. It provides you with a bird’s-eye view of the entire request flow, allowing you to pinpoint bottlenecks, latency issues, and even failures.
If you're managing a microservices-based architecture, distributed tracing is essential for troubleshooting and performance optimisation.
Istio’s Role in Distributed Tracing
So, where does Istio fit into all this?
Istio plays a critical role by injecting tracing headers into every service-to-service communication. Its Envoy proxy automatically captures trace data, meaning you don’t have to manually instrument each service. Istio handles the heavy lifting, collecting the information you need to understand the performance of your entire service mesh. This data can then be forwarded to your chosen distributed tracing system, like Jaeger or Zipkin.
TraceID and SpanID
Let’s break it down simply. Every request that travels through your microservices architecture generates a TraceID—a unique identifier that ties together all the individual service calls involved in handling that request.
Think of it as the overarching identifier for the entire transaction.
Each service call within that trace also gets its own SpanID, representing a single unit of work, such as querying a database or calling another service.
By connecting the dots between these TraceIDs and SpanIDs, Istio tracing gives you a clear picture of what’s happening across your microservices. It’s like having a detailed map of every stop a request makes as it travels through your architecture.
Now that you have the foundational knowledge of Istio tracing let's dive into how to set up tracing in Istio and start making sense of your distributed systems.
Configuring Tracing in Istio
Ready to get Istio tracing up and running?
The good news is that configuring tracing in Istio is straightforward, and you can start tracking requests across your microservices architecture in no time.
Let's walk through the essential steps to enable tracing in Istio and ensure you're capturing the data that matters most.
Step 1: Enabling Tracing in Istio
First, you'll need to enable tracing within Istio. By default, Istio’s Envoy proxy supports tracing, but you must ensure it’s properly configured.
Begin by setting the values.pilot.traceSampling parameter in your Istio configuration to control the sampling rate. A 100% rate is ideal for testing but may not be practical in production due to data volume.
Most production environments go for a lower sampling rate, like 1-5%, to balance performance and visibility.
You can configure this via the IstioOperator configuration file or Helm charts. Here’s a basic example of enabling tracing in your Istio configuration:
apiVersion: install.istio.io/v1alpha1 |
Step 2: Envoy Proxy and Tracing Headers
Once tracing is enabled, Istio’s Envoy proxy takes care of generating and propagating tracing headers as requests pass through the service mesh. Envoy injects tracing headers like x-request-id, x-b3-traceid, x-b3-spanid, and x-b3-sampled into each request.
These headers track the journey of each request across different services.
Envoy’s automatic header propagation simplifies tracing by ensuring every service in the chain adds its own span to the trace, giving you a detailed breakdown of each step.
For developers looking for deeper analysis of logs and traces, tools like OpenObserve can be a powerful complement. OpenObserve is designed to handle large-scale log ingestion and trace data, making it a cost-effective alternative to Datadog, Elasticsearch, or Splunk for monitoring your Istio traces in real time. Sign up now to get started!
Step 3: Required Headers for Tracing
To ensure full trace propagation, your services must properly handle the required tracing headers. Envoy automatically generates and propagates the following key headers:
- x-request-id: A unique identifier for the request.
- x-b3-traceid: The TraceID that links all spans in a single trace.
- x-b3-spanid: A unique identifier for each span.
- x-b3-sampled: Indicates if the trace is being sampled or not (useful for controlling data volume).
Make sure that your services are passing these headers between them. If you’re using a language that doesn’t automatically propagate these headers, you may need to modify your application code to forward them.
With Istio tracing set up and the necessary headers in place, the next step is understanding how trace context is propagated between services to ensure seamless tracking across your microservices.
Propagation of Trace Context
Getting Istio tracing up and running is only the beginning. Ensuring that trace headers are properly propagated between your services is what guarantees complete visibility across your microservices. But this can be a common stumbling block for developers.
If the headers aren’t passed correctly, your traces will have gaps, making it hard to diagnose issues or understand the flow of requests.
Let’s break down what you need to know to get it right with practical tips to help you avoid common mistakes.
Passing Tracing Headers Between Services
Once Istio injects the initial trace headers, your services need to keep passing those headers along as they communicate. Without this, each service will generate its own trace, and you’ll lose the complete picture of a request's journey through your system.
So, what exactly needs to happen?
Your services must pass along the following critical headers:
- x-request-id: A unique identifier for each request, which helps tie all services into the same trace.
- x-b3-traceid: The ID that binds all spans together for the same trace.
- x-b3-spanid: A unique identifier for each individual span in the trace.
- x-b3-sampled: Indicates whether this trace is being sampled (which can affect how much data is collected).
Implementing Header Propagation in Your Code
Not all frameworks or languages automatically handle header propagation. You must ensure your code is set up to pass along these tracing headers. Here's how you can implement this:
Check Your Framework’s Default Behavior: Some popular frameworks like Spring Boot or Flask automatically forward tracing headers, but not all do. Be sure to verify your framework’s behaviour regarding tracing.
Modify Application Code: If your services aren’t automatically forwarding the necessary headers, you’ll need to add that functionality.
For example, in Python using Flask, you could manually extract and forward the headers:
import requests |
Test the Propagation: Once implemented, test it to ensure that the tracing headers are correctly passed along. You can monitor this by checking your distributed tracing system (like OpenObserve) to confirm the headers are consistent across services.
Avoiding Common Pitfalls
A common mistake is forgetting to forward headers in every service. One missed service and the trace breaks. It’s crucial to ensure that all services are aware of the tracing headers and forward them consistently. Another issue developers run into is modifying the headers incorrectly, which can disrupt the trace context.
Proper propagation of trace headers is the backbone of a solid Istio tracing setup. Now that you've ensured trace headers are being handled correctly, it’s time to explore the different distributed tracing systems supported by Istio and how they can fit into your observability stack.
Distributed Tracing Systems Supported by Istio
When you’ve got Istio tracing set up, the next step is choosing the right distributed tracing system to collect and visualise your trace data. Fortunately, Istio supports several powerful tools, each offering its own strengths depending on your needs.
Jaeger
Jaeger is one of the most popular distributed tracing systems and a natural fit for Istio tracing. Known for its rich feature set and scalability, Jaeger is widely adopted across industries.
To configure Istio with Jaeger, you must install Jaeger in your cluster and set up the tracing.jaeger settings in Istio’s configuration.
apiVersion: install.istio.io/v1alpha1 |
Once configured, Jaeger will start collecting traces from Istio, allowing you to visualise them through Jaeger's UI.
Zipkin
Zipkin is another great option, especially if you’re looking for something lightweight. While it’s similar to Jaeger in terms of core functionality, Zipkin offers faster deployment and can be a bit easier to set up. To integrate Zipkin with Istio, you’ll modify the IstioOperator configuration just as you did with Jaeger, but specify tracer: "zipkin".
apiVersion: install.istio.io/v1alpha1 |
Zipkin’s flexibility and ease of use make it a good option if you’re looking for a simple but effective tracing system.
OpenTelemetry
OpenTelemetry is quickly becoming the industry standard for observability, as it supports traces, metrics, and logs in one unified platform. If you're thinking about future-proofing your observability stack, Istio tracing with OpenTelemetry is a great investment.
To configure OpenTelemetry with Istio, you'll need to deploy the OpenTelemetry Collector in your cluster and configure Istio to send traces there:
apiVersion: install.istio.io/v1alpha1 |
This setup ensures that OpenTelemetry collects all the trace data from your services, with the added benefit of handling metrics and logs as well.
Apache SkyWalking
Apache SkyWalking is an open-source performance monitoring system that offers tracing, logging, and metrics collection—making it another great option for Istio users. SkyWalking is particularly well-suited for teams that want an all-in-one tool with minimal configuration.
To get SkyWalking working with Istio, you’ll follow a similar setup, specifying tracer: "skywalking" in your configuration.
apiVersion: install.istio.io/v1alpha1 |
SkyWalking's versatility makes it an attractive option for organisations looking for a single tool to monitor multiple aspects of their system.
OpenObserve
If you're looking for a unified observability platform but want to keep storage costs low, OpenObserve might be your ideal choice. OpenObserve supports logs, traces, and metrics in one place, offering up to 140x lower storage costs compared to systems like Elasticsearch. This makes it a strong contender for teams using Istio who need comprehensive observability without the high price tag.
While not natively integrated with Istio, OpenObserve can easily collect trace data by setting it up as a logging and tracing backend using its powerful APIs. This allows you to centralise all your logs and traces for easier analysis and decision-making.
Head over to OpenObserve’s website to explore how to monitor your microservices with logs, metrics, and traces—all in one platform.
Read more about Revolutionizing Observability - Unveiling OpenObserve, the High-Performance, Cloud-Native Platform
Now that you have a solid understanding of the tracing systems supported by Istio, let's examine how to fine-tune your tracing with sampling strategies.
Sampling Strategies
When you're working with Istio tracing, one of the key decisions you'll make is how much trace data to collect. While capturing every trace might sound tempting, it can quickly overwhelm your system, consume resources, and drive up storage costs.
This is where trace sampling comes in, helping you balance between full visibility and system performance.
What is Trace Sampling?
In simple terms, trace sampling refers to the practice of capturing a subset of all possible traces. Instead of recording every single transaction passing through your microservices, you configure Istio to sample a certain percentage.
This allows you to control the amount of trace data generated, ensuring your system remains performant without losing critical insights.
When to Use Low vs. High Sampling Rates
Different scenarios call for different sampling rates, and understanding when to use a low or high rate can make all the difference in monitoring your system effectively.
- Low Sampling Rate (1-5%) for Performance Monitoring:
If your primary goal is to monitor the overall health and performance of your microservices, a low sampling rate is usually sufficient. This helps reduce the data overhead while still providing enough traces to detect patterns, bottlenecks, or emerging issues.
For example, in a stable production environment where your services run smoothly, setting the sampling rate to 1% ensures you’re not drowning in unnecessary data but can still keep tabs on performance.
- High Sampling Rate (50-100%) for Debugging:
When you’re troubleshooting or debugging a specific service, increasing the sampling rate can be a lifesaver. Capturing more traces gives you a deeper look into the interactions between services, making it easier to pinpoint the exact source of issues.
Imagine you’re dealing with a sporadic latency problem in one of your core services; setting the sampling rate to 100% temporarily ensures you don’t miss any data that could be critical in solving the problem.
Setting and Modifying the Sampling Rate in Istio
Configuring the sampling rate in Istio is straightforward. By default, Istio sets the sampling rate to 1%, but you can easily modify it based on your needs.
Here’s how you can adjust the sampling rate using the IstioOperator configuration:
apiVersion: install.istio.io/v1alpha1 |
In this example, the sampling rate is set to 50%, meaning half of all requests will be traced. Adjust this based on whether you're monitoring the system for performance or debugging a specific issue.
Best Practices for Sampling Configuration
- Start with a Low Sampling Rate: In most production environments, starting with a 1-5% sampling rate will keep things manageable without overloading your system. This is often enough to monitor general trends and catch issues before they escalate.
- Adjust Based on System Needs: During peak load times or when scaling up your microservices, it may be wise to reduce the sampling rate further to avoid unnecessary resource consumption. On the other hand, when rolling out new features or troubleshooting, temporarily increasing the rate can give you the detailed data you need.
- Dynamic Sampling: Some advanced observability setups use dynamic sampling, adjusting the rate based on specific criteria like error rates or performance degradation. This approach lets you collect more traces when your system is under stress while reducing the load during normal operation.
Sampling is the key to maintaining a balance between performance and trace visibility in Istio tracing. Now, let's move on to how to troubleshoot common tracing issues and avoid potential pitfalls.
Troubleshooting Common Issues
Even with Istio tracing set-up, things don’t always run smoothly. Missing traces, misconfigured services, and mysterious gaps in your data can leave you scratching your head.
The good news?
Most of these issues are common, and there are clear ways to resolve them.
Let’s dive into the most frequent pitfalls and how you can troubleshoot them efficiently.
Missing Traces? Start Here
One of the most frustrating issues you’ll face is missing traces. You’ve got everything configured, yet some requests just don’t appear in your tracing system.
The first thing to check is whether your sampling rate is set too low. If it’s set at 1%, for instance, you’ll only capture a fraction of the traces, which can make it seem like traces are missing. Increasing the rate temporarily to 100% can help confirm whether this is the issue.
Next, ensure that the tracing headers are being properly propagated between your services. Use the following command to check if the headers are making their way across:
kubectl logs <pod-name> -n <namespace> | grep 'x-b3-traceid' |
If you don’t see any trace IDs in the logs, it’s likely that the headers aren’t being passed along correctly.
Double-Check Port Naming Conventions
Istio requires strict adherence to port naming conventions, and this is a common source of problems. If your ports aren’t named correctly, Istio might not inject the necessary sidecar proxies, and as a result, you won’t capture traces for those services.
The solution?
Ensure that service ports are named with a protocol prefix like http-, grpc-, or tcp-.
For example:
ports: |
Incorrect naming here can break tracing across the entire service mesh, so it’s worth double-checking.
Validate Namespace Labeling
Another common mistake is overlooking the need for correct namespace labeling. Istio’s tracing features only work if the namespace has the appropriate labels.
To ensure the correct labels are applied, run:
kubectl label namespace <namespace> istio-injection=enabled |
This command enables Istio’s sidecar injection for the namespace, ensuring all services within it are properly instrumented for tracing.
Configuration Pitfalls to Avoid
- Misconfigured Mesh Policies: If you’re running into issues with Istio’s service mesh policies, such as mTLS settings, tracing can break due to miscommunication between services. Make sure the mesh policies are consistent and allow proper communication between services.
- Third-Party Tracing Platform Misconfiguration: If you’re using third-party tracing platforms like Jaeger or Zipkin, double-check that Istio is properly configured to send traces to those backends. Misconfigured endpoints or ports can result in traces not reaching the backend at all.
To verify that traces are reaching your tracing system, you can run a port-forward command for Jaeger:
kubectl port-forward svc/jaeger-query 16686:16686 -n istio-system |
Then access Jaeger via http://localhost:16686 to confirm that the traces are flowing in.
Debugging Commands to Validate Trace Context Propagation
If traces aren’t showing up in the backend, here’s a quick way to validate if trace context is being propagated across services:
Check for Sidecar Injection:
kubectl get pods -n <namespace> -o=jsonpath="{.items\[*].metadata.annotations\['sidecar.istio.io/status']}" |
This checks whether the sidecar proxies are correctly injected into your pods.
Monitor Trace Flow:
If you're not seeing trace context propagate, inspect the logs of your services for the trace headers. Use the earlier kubectl logs command to ensure that headers like x-b3-traceid are present throughout the flow.
With these troubleshooting steps, you can easily resolve the most common Istio tracing issues.
Read more about Jidu's Journey to 100% Tracing Fidelity with OpenObserve. A Case Study
Advanced Configuration and Customization
Once you’ve got Istio tracing up and running, you may find that out-of-the-box settings don’t quite meet all your needs. Whether you're looking to customise trace behaviour, experiment with advanced propagation techniques, or explore new standards like W3C Trace Context, the good news is that Istio allows for significant flexibility.
Let’s dive into some real-world customisation strategies to enhance your tracing setup.
Tailoring Tracing Configurations to Your Needs
While the default tracing configuration in Istio works well for most setups, you might want to fine-tune it for specific use cases.
For example, you can customise which requests get traced by using custom trace sampling rules or adjusting the headers being propagated.
One common customisation is adding custom headers to enrich your traces.
For instance, you may want to add a user ID or a session ID to your trace data to better track specific user flows across your services. You can achieve this by modifying your application to include the additional headers when propagating trace information:
# Add custom headers in your application |
By adding these custom headers, you gain more granularity and can troubleshoot issues at the user or session level.
Exploring Advanced Propagation Techniques
Sometimes the basic trace propagation methods may not be enough, especially when dealing with complex microservices environments or external systems. One advanced technique is to implement trace context propagation across multiple service meshes or across services that don’t natively support Istio. This can be useful when you need to integrate with third-party services or legacy systems.
In these cases, you may need to manually forward the trace headers or even transform them if the external system expects different formats. By writing custom middleware or hooks, you can ensure that trace context is preserved, even across different environments.
Another helpful technique is head-based sampling, which allows you to make sampling decisions based on the content of the request headers before it even enters your mesh.
This technique is particularly helpful when you want to prioritise tracing for certain types of requests, such as high-value transactions.
Looking Forward: W3C Trace Context
The future of tracing is being shaped by emerging standards like the W3C Trace Context, which aims to create a unified format for tracing headers across all platforms and tools. Adopting this standard ensures interoperability between various tracing systems and allows for easier integration with third-party services.
To start using W3C Trace Context in your Istio tracing setup, you can enable support by configuring your Envoy proxy to handle the new header format:
apiVersion: install.istio.io/v1alpha1 |
By enabling this feature, you ensure that your services are future-proofed and ready to integrate seamlessly with any observability tool that supports the W3C standard.
For developers looking to dive deeper into W3C Trace Context and other advanced tracing configurations, check out the official W3C Trace Context documentation.
Advanced tracing configurations allow you to fine-tune your observability stack, making it more resilient, scalable, and adaptable to your specific use cases.
Conclusion
By now, you've seen how powerful Istio tracing can be in monitoring and optimising your microservices architecture. Whether you're configuring basic traces, customising for advanced use cases, or exploring the future of tracing with W3C Trace Context, Istio provides the flexibility to adapt to your needs.
For those looking to streamline their observability further, consider using OpenObserve (O2). With support for logs, metrics, and traces all in one platform, OpenObserve offers a cost-effective alternative to traditional tools like Elasticsearch and Datadog. It’s easy to get started, and its simple setup can save you time and significantly reduce storage costs.
Ready to level up your monitoring? Sign up here or explore more on OpenObserve’s website. You can also check out the code and contribute via GitHub.